PyFlyt/QuadX-Pole-Balance-v3
#
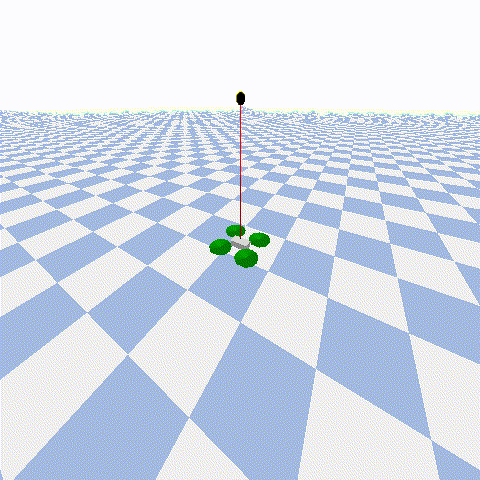
Task Description#
The goal of this environment is to hover a quadrotor drone for as long as possible while balancing a 1 meter long pole.
Usage#
import gymnasium
import PyFlyt.gym_envs
env = gymnasium.make("PyFlyt/QuadX-Pole-Balance-v3", render_mode="human")
term, trunc = False, False
obs, _ = env.reset()
while not (term or trunc):
obs, rew, term, trunc, _ = env.step(env.action_space.sample())
Environment Options#
- class PyFlyt.gym_envs.quadx_envs.quadx_pole_balance_env.QuadXPoleBalanceEnv(sparse_reward: bool = False, flight_mode: int = -1, flight_dome_size: float = 3.0, max_duration_seconds: float = 20.0, angle_representation: Literal['euler', 'quaternion'] = 'quaternion', agent_hz: int = 40, render_mode: None | Literal['human', 'rgb_array'] = None, render_resolution: tuple[int, int] = (480, 480))#
Simple Hover Environment with the additional goal of keeping a pole upright.
Actions are direct motor PWM commands because any underlying controller introduces too much control latency. The target is to not crash and not let the pole hit the ground for the longest time possible.
- Parameters:
sparse_reward (bool) – whether to use sparse rewards or not.
flight_mode (int) – the flight mode of the UAV
flight_dome_size (float) – size of the allowable flying area.
max_duration_seconds (float) – maximum simulation time of the environment.
angle_representation (Literal["euler", "quaternion"]) – can be “euler” or “quaternion”.
agent_hz (int) – looprate of the agent to environment interaction.
render_mode (None | Literal["human", "rgb_array"]) – render_mode
render_resolution (tuple[int, int]) – render_resolution.